Introduction
A class diagram is a static type of Unified Modeling Language (UML) diagram that visually represents the structure of a system by showing its classes, attributes, operations, and relationships between objects. It serves as a blueprint for object-oriented software design, providing a clear and concise way to understand and document the architecture of a system.
Purpose and Functionality
Visualizing System Structure
Class diagrams help developers understand and document the structure of a system by showing how different classes interact and relate to each other. This visual representation is crucial for designing robust and maintainable software systems.
Modeling Software
Class diagrams enable the modeling of software at a high level of abstraction, allowing developers to focus on the design without delving into the source code. This abstraction helps in identifying potential issues early in the development process.
Object-Oriented Design
Class diagrams are fundamental to object-oriented modeling. They outline the building blocks of a system and their interactions, making it easier to implement object-oriented principles such as encapsulation, inheritance, and polymorphism.
Data Modeling
Class diagrams can also be used for data modeling, representing the structure and relationships of data within a system. This is particularly useful in database design, where entities and their relationships need to be clearly defined.
Blueprint for Code
Class diagrams serve as a blueprint for constructing executable code for software applications. They provide a clear roadmap for developers, ensuring that the implementation aligns with the designed architecture.
Key Components
Classes
Classes are represented by rectangles divided into three sections:
- Class Name: The top section contains the name of the class.
- Attributes: The middle section lists the attributes or data members that define the state of the class.
- Operations (Methods): The bottom section lists the operations or functions that the class can perform.
Relationships
Relationships between classes are shown using lines and symbols:
- Generalization: Represents inheritance, where a class (subclass) inherits attributes and operations from another class (superclass). It is depicted by a hollow arrowhead pointing from the subclass to the superclass.
- Aggregation: Indicates that one class contains instances of another class, but the contained class can exist independently. It is depicted by a hollow diamond at the end of the line connected to the containing class.
- Composition: A stronger form of aggregation where the contained class cannot exist without the containing class. It is depicted by a filled diamond at the end of the line connected to the containing class.
- Association: Represents a relationship between two classes, indicating that one class uses or interacts with another. It is depicted by a solid line connecting the two classes.
Example Diagrams using PlantUML
Basic Class Diagram
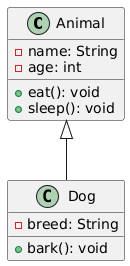
Diagram with Aggregation and Composition
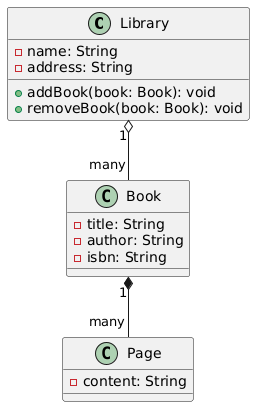
Diagram with Association

Example – Order system
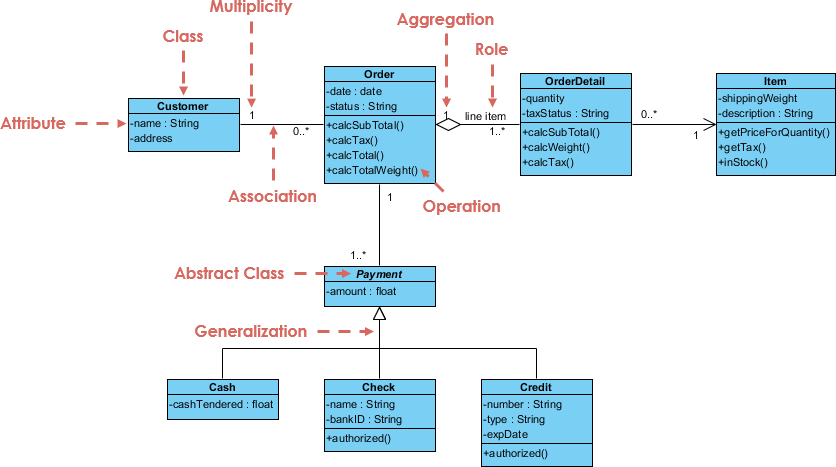
Key Elements
-
Classes:
- Customer: Represents the customer placing the order.
- Attributes:
name
(String), address
(String).
- Order: Represents the order placed by the customer.
- Attributes:
date
(Date), status
(String).
- Operations:
calcSubTotal()
, calcTax()
, calcTotal()
, calcTotalWeight()
.
- OrderDetail: Represents the details of each item in the order.
- Attributes:
quantity
(int), taxStatus
(String).
- Operations:
calcSubTotal()
, calcWeight()
, calcTax()
.
- Item: Represents the items being ordered.
- Attributes:
shippingWeight
(float), description
(String).
- Operations:
getPriceForQuantity()
, getTax()
, inStock()
.
- Payment (Abstract Class): Represents the payment for the order.
- Attributes:
amount
(float).
- Cash: Subclass of Payment, represents cash payments.
- Attributes:
cashTendered
(float).
- Check: Subclass of Payment, represents check payments.
- Attributes:
name
(String), bankID
(String), isAuthorized
(boolean).
- Credit: Subclass of Payment, represents credit card payments.
- Attributes:
number
(String), type
(String), expDate
(Date), isAuthorized
(boolean).
-
Relationships:
- Association:
- Customer and Order: A customer can place multiple orders (
0..*
multiplicity on the Order side).
- Order and OrderDetail: An order can have multiple order details (
1..*
multiplicity on the OrderDetail side).
- OrderDetail and Item: Each order detail is associated with one item (
1
multiplicity on the Item side).
- Aggregation:
- Order and OrderDetail: Indicates that OrderDetail is a part of Order, but OrderDetail can exist independently.
- Generalization:
- Payment and its subclasses (
Cash
, Check
, Credit
): Indicates inheritance, where Cash, Check, and Credit are specific types of Payment.
- Role:
- OrderDetail and Item: The role
line item
indicates the specific role of OrderDetail in the context of an Order.
-
Multiplicity:
- Indicates the number of instances of one class that can be associated with a single instance of another class. For example, a Customer can place multiple Orders (
0..*
).
-
Abstract Class:
- Payment: Marked as an abstract class, meaning it cannot be instantiated directly and serves as a base class for other payment types.
Explanation
- Customer: Represents the entity placing the order, with basic attributes like name and address.
- Order: Represents the order itself, with attributes like date and status, and operations to calculate subtotal, tax, total, and total weight.
- OrderDetail: Represents the details of each item in the order, including quantity and tax status, with operations to calculate subtotal, weight, and tax.
- Item: Represents the items being ordered, with attributes like shipping weight and description, and operations to get price for quantity, tax, and stock status.
- Payment: An abstract class representing the payment for the order, with an attribute for the amount. It has subclasses for different payment methods:
- Cash: Represents cash payments with an attribute for the cash tendered.
- Check: Represents check payments with attributes for the name, bank ID, and authorization status.
- Credit: Represents credit card payments with attributes for the card number, type, expiration date, and authorization status.
The diagram effectively captures the structure and relationships within an order processing system, providing a clear visual representation of how different components interact.
Conclusion
Class diagrams are an essential tool in UML modeling, providing a clear and structured way to represent the architecture of a system. By understanding the key components and relationships, developers can create robust and maintainable software designs. Using tools like PlantUML, these diagrams can be easily visualized and shared among team members, enhancing collaboration and ensuring a consistent understanding of the system’s structure.
References
-
Visual Paradigm Online Free Edition:
- Visual Paradigm Online (VP Online) Free Edition is a free online drawing software that supports Class Diagrams, other UML diagrams, ERD tools, and Organization Chart tools. It features a simple yet powerful editor that allows you to create Class Diagrams quickly and easily. The tool offers unlimited access with no restrictions on the number of diagrams or shapes you can create, and it is ad-free. You own the diagrams you create for personal and non-commercial use. The editor includes features such as drag-to-create shapes, inline editing of class attributes and operations, and a variety of formatting tools. You can also print, export, and share your work in different formats (PNG, JPG, SVG, GIF, PDF) 123.
-
Impressive Drawing Features:
- Visual Paradigm Online provides advanced formatting options to enhance your diagrams. You can position shapes precisely using alignment guides and format your Class Diagrams with shape and line formatting options, font styles, rotatable shapes, embedded images and URLs, and shadow effects. The tool is cross-platform compatible (Windows, Mac, Linux) and can be accessed through any web browser. It also supports Google Drive integration for seamless saving and accessing of your diagrams 23.
-
Comprehensive Diagramming Options:
- Visual Paradigm Online supports a wide range of diagram types, including UML diagrams (class, use case, sequence, activity, state, component, and deployment diagrams), ERD tools, Organization Charts, Floor Plan Designers, ITIL, and Business Concept Diagrams. The tool is designed to be easy to use, with drag-and-drop functionality and smart connectors that snap into place. It also offers a rich set of formatting options, including over 40 connector types and various paint options 45.
-
Learning and Customization:
- Visual Paradigm provides an easy-to-use platform for creating and managing class diagrams, making it an excellent choice for software developers and engineers. You can customize your class diagrams by changing colors, fonts, and layout. The tool also supports creating relationships between classes, such as associations, inheritance, and dependencies. Visual Paradigm is a powerful UML modeling tool that helps in representing the static structure of a system, including the system’s classes, their attributes, methods, and the relationships between them 67.
-
Community and Support:
- Visual Paradigm Community Edition is a free UML software that supports all UML diagram types. It is designed to help users learn UML faster, easier, and quicker. The tool is intuitive and allows you to create your own Class Diagrams with ease. Visual Paradigm is trusted by over 320,000 professionals and organizations, including small businesses, Fortune 500 companies, universities, and government sectors. It is used to prepare the next generation of IT developers with the specialized skills needed for the workspace 89.
These references highlight the comprehensive features and benefits of using Visual Paradigm for creating class diagrams, making it a recommended tool for both individual and professional use.